- Coding Beauty
- Posts
- Promise.all() vs Promise.allSettled() in JS: The little-known difference
Promise.all() vs Promise.allSettled() in JS: The little-known difference
Vowel removals, Promise confusion, and more
Want SOC 2 compliance without the Security Theater?
Get the all-in-one platform for SOC 2
Build real-world security đź’Ş
Penetration testing, compliance software, 3rd party audit, & vCISO
Featured content
Techpresso: a daily rundown of what’s happening in tech and what you shouldn’t miss. Read by professionals at Google, Apple, and OpenAI. Check it out
GPTE AI: Discover over 5,000 new AI tools for productivity, design, content creation, and more. Check it out
I can't believe some developers think they are interchangeable.
They are not.
all()
and allSettled()
are much more different than you think.
So let's say I decided to singlehandedly create my own ambitious new music app to finally take down Spotify.
I've worked tirelessly to make several music deals and have all the backend data ready.
Now I'm creating the app's homepage: a shameless carbon copy of Spotify showing off daily mixes, recently played, now playing, and much more.

All this data will come from different API URLs.
Of course I wouldn’t be naive enough to fetch from all the URLs serially and create a terrible user experience (or would I?)
async function loadHomePage() {
const apiUrl = 'music.tariibaba.com/api';
const dailyMixesUrl = `${apiUrl}/daily`;
const nowPlayingUrl = `${apiUrl}/nowPlaying`;
// ❌ Serial loading
const dailyMixes = await (
await fetch(dailyMixesUrl)
).json();
const nowPlaying = await (
await fetch(nowPlayingUrl)
).json();
return { dailyMixes, nowPlaying };
}
No I wouldn’t.
So instead I use use Promise.all()
to fetch from all these URLs at once:
Much better, right?
async function loadHomePage() {
const apiUrl = 'music.tariibaba.com/api';
const dailyMixesUrl = `${apiUrl}/daily`;
const nowPlayingUrl = `${apiUrl}/nowPlaying`;
// Promise.all() ??
await Promise.all([
fetch(dailyMixesUrl),
fetch(nowPlayingUrl),
]);
return { dailyMixes, nowPlaying };
}
Wrong.
I just made the classic developer error of forgetting to handle the error state.
Let me tell you something: When Promise.all()
fails, it fails:
async function loadHomePage() {
const apiUrl = 'music.tariibaba.com/api';
const dailyMixesUrl = `${apiUrl}/daily`;
const nowPlayingUrl = `${apiUrl}/nowPlaying`;
// Promise.all() ??
try {
await Promise.all([
fetch(dailyMixesUrl),
fetch(nowPlayingUrl),
]);
} catch (err) {
// ❌ Which failed & which succeeded? We have no idea
console.log(`error: ${err}`);
}
return { dailyMixes, nowPlaying };
}
What do you when one of those requests fail? Am I just going to leave that UI section blank and not try again?
But look what Promise.allSettled()
would have done for us:
async function loadHomePage() {
const apiUrl = 'music.tariibaba.com/api';
const dailyMixesUrl = `${apiUrl}/daily`;
const nowPlayingUrl = `${apiUrl}/nowPlaying`;
// ...
// Promise.all() ??
try {
const promises = await Promise.allSettled([
fetch(dailyMixesUrl),
fetch(nowPlayingUrl),
// ...
]);
const succeeded = promises.filter(
(promise) => promise.status === 'fulfilled'
);
const failed = promises.filter(
(promise) => promise.status === 'rejected'
);
// âś… now retry failed API requests until succeeded
} catch (err) {
// We don't need this anymore!
console.log(`error: ${err}`);
}
return { dailyMixes, nowPlaying };
}
Now I could easily set up a clever system to tirelessly retry all the failed requests until they all work:
73%.
Did you know that 73% of developers worldwide rely on the same code editor?
Yes, the 2023 Stack Overflow Developer Survey results are in, and yet again, Visual Studio Code was by far the most used development environment.

And we all know why: it’s awesome.
But are we fully exploring its potential? In this article, we unfold some compelling VS Code features that enhance productivity with local source control, animated typing, and rapid line deletion, amongst others. Let us start using them to achieve our coding goals faster than ever.
1. Timeline view: local source control
The Timeline view gives us local source control.
Many of us know how useful Git and other source control tools are, helping us easily track file changes and revert back to a previous point when needed.
So the Timeline view in VS Code provides an automatically updated timeline of important events related to a file, such as Git commits, file saves, and test runs.

Expand this view to see a list of snapshot of events related to the current file. Here it’s file saves, but also Git commits where the file was staged.

Hover over the snapshot item to view the date and time when VS Code made the snapshot.

Select a snapshot item to see a diff view showing the changes between the file at the snapshot time and the file presently.

2. Autosave: no more Ctrl + S
Can you count how many times you’ve used this shortcut? You probably do it unconsciously now.
The Autosave feature automatically saves files as we work on them, removing the need for manual saving. With autosave, we eliminate Ctrl + S fatigue, save time, and gain certainty of always working with the latest changes to the files.
It’s not perfect though, and it’s up to you to weigh the pros and cons – which we comprehensively cover here.

No autosave
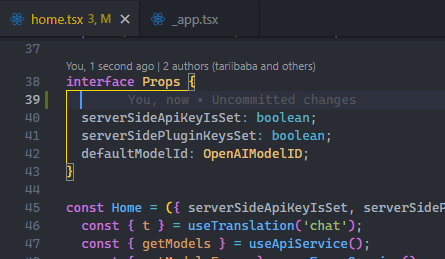
Autosave enabled — the unsaved indicator no longer shows
Use File > Auto Save to enable the feature easily.

Thanks for taking the time to read today’s issue.
Don’t let the bugs byte,
Tari Ibaba